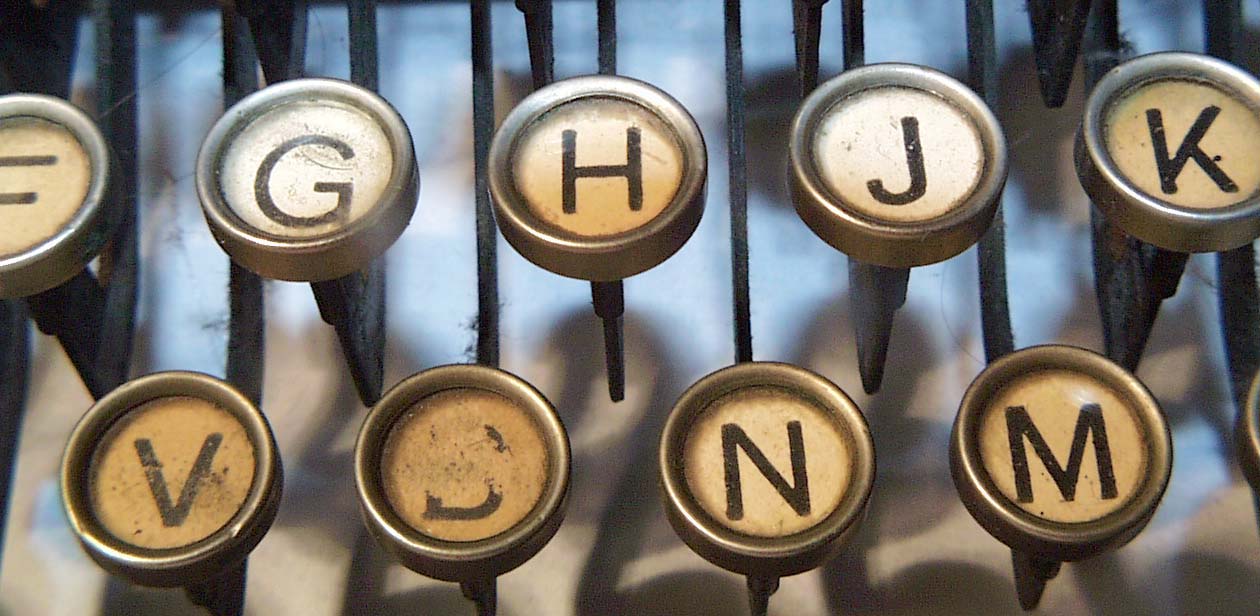
You obviously must have learned about ASCII codes while going through any programming courses. This is because one obvious question you'd see would be to input a character and output the corresponding ASCII code of that character. Or the question would be the exact opposite or maybe it would be to display an ASCII table. So today, we're going to take a look at that easy little program to input a character and to display the corresponding ASCII for that.
Before we take a look at that program, I need to tell you a few things about ASCII itself and how people normally misunderstands the concept itself. I must admit that I too didn't knew much about ASCII until I started to think about the lack of perfection that would exist once I code it. And that led to me to the doors of new knowledge that I didn't knew before. So, a lot of thanks for that.
ASCII & the Myths

ASCII codes were created for the alphabets, A-Z and a-z as well as for the numbers 0-9. But that's not just it. ASCII also contains several other codes for other characters such as various punctuators. These are print characters and ASCII also contains non-print control characters which control the text as such and are very much deprecated in use nowadays.
Later, an extended ASCII character set was introduced containing 256 characters. So, that's basically about ASCII.
Most of you (like me) would'e thought at first that everything you see in the keyboard would also be a part of the ASCII or the keyboard follows the ASCII scheme. Thus, there would be codes for arrow keys and some other keys. And it could be the ASCII scheme that helps developers use arrow keys for control in a program -- use the codes accordingly and run a game or navigate using these keys. Every key should have an ASCII code.
And that's entirely wrong. Keyboards are not based on ASCII. Moreover, the control keys such as arrow-keys are not a part of the ASCII at all. That means there exist no ASCII code for these keys. Although ASCII was defined before even C was developed, it doesn't restrict these languages to use an alternative method.
But there must exist some standard that identifies the keyboard as well, right? Well, there exists one which is OS specific. Each OS has its own predefined set of codes that identifies these keys. And this shouldn't be ASCII exactly. Windows has its own set and Linux has its own one. Or so I understood. And for that, these OSs and the programs define this under specific libraries and that's how it all works.
In a nutshell, ASCII is limited to mainly typographic characters. It doesn't represent Keyboard keys and that is represented by another scheme which is basically OS specific when it comes down to application level stuff. BIOS also have a representation of these characters.
Back to our program. Now that you know everything about ASCII, you also know some characters of the keyboard can't be represented. And this brings some limitations to our program which I mostly tried to overcome but couldn't exceed due to my knowledge limits and for your better understanding. So, grab the source code below and then let us analyze.
Source Code
#include <iostream> #include <conio.h> using namespace std; void main() { char ch; cout << "\nEnter the character: "; ch = _getch(); //cout << ch; cout << "\nThe ASCII code is: " << (int)ch; _getch(); }
Analysis
The program is straight-forward. Input a character and then type-cast the character variable ch to an int value which would be the corresponding ASCII code. Notice that I (deliberately) used the conio.h library of VC++ and the _getch() function which is just a revamped getch() function.
Why didn't I use cin? Why not cin.get()? The first one -- simply because it ignores white-spaces. cin.get() was more preferable but it still would use a new-line as the delimiter and even if the delimiter was changed, the delimiter value would remain in the buffer. It would read normal white-space but not the new-line and I couldn't find a suitable delimiter. _getch() even though is not a part of the standard library and is actually one that belonged to the old Turbo C++ libraries is quite suitable because of its function. It doesn't wait for a delimiter and reads exactly one key and skips to the next-code. Both cin and cin.get() would fail there and it would be quite cumbersome (not really) to type in and then press the enter key.
Try running the program and enter some sample characters. Most would work fine until you try pressing the arrow-keys or function-keys. For me, an output appeared but the output window closed immediately which should not be happening unless I pressed some key. And if you display the output again, you can see the values that was printed just before the exit.
For me, all the arrow keys bore a value of -32 and the function keys being 0. I don't know why this appears or what caused the program to exit but that's all I know.
I hope the program was readable. You can use cin and cin.get() but keep in mind the limitations. And if you got a better method or more info on this topic, be sure to comment below. I'm just an intermediate but I still would love to hear the new info.
Sources: Wikipedia, StackOverflow (1) (2), ASCIITABLE